Secure Image Uploading on Server Using PHP
25-09-2017
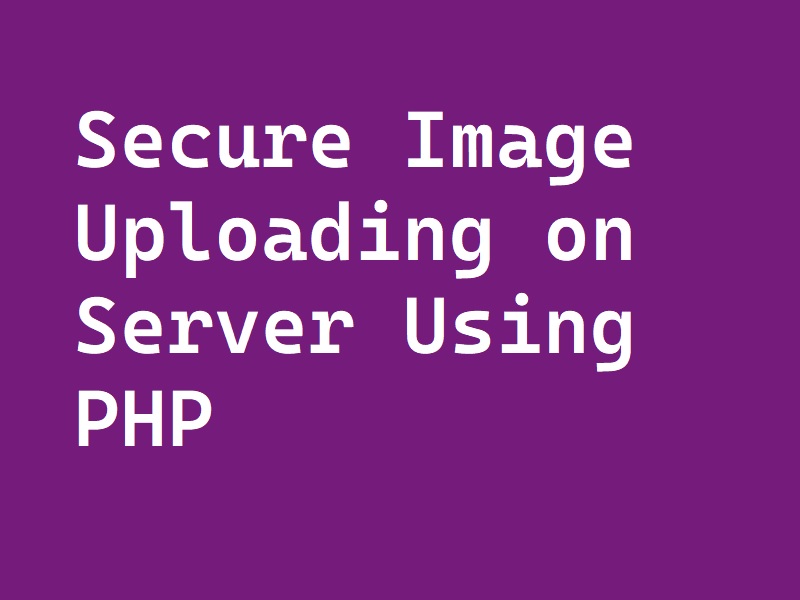
Upload the Image file on the server using a secure way. While uploading images on the server, the Developer must take care of certain security so that attackers would not upload any infected miscellaneous files that contain the virus.
In PHP, File uploading is the most sensitive part when it comes to security. When a developer allows a user to upload a file, validation must be done in such a way that only allowed file types should be uploaded to the server. If validation is not done in the right way, an attacker can upload any type of file on the server and it will ruin your web application. For example, an attacker may upload a PHP file that can display each file and its contents on the server. An attacker can write a code in the PHP file to view all user's credentials and can log in to any user account, OR can Delete the entire database, anything can happen.
Security Key Points of Validation While Uploading File
Here, i'm listing the Security Key Points of Validation While Uploading File.- Check File Extension : This is very first point to be checked. We make sure first that the uploaded file has the certain extension that we are allowing.
- Check File MIME Content-Type : Uploaded file having the certain allowed extension cannot validate the file type. For example, Uploaded file content contain PHP file but can have extension .jpg. Using MIME, we can validate the content of the file and make sure the uploaded file is valid.
- Restrict File Size : It is good technique to restrict the size of file. Otherwise, the user can upload any size of a file multiple times and full our server drive.
Imgae Uploading Code Demo With Sample Code Download
<form action="" method="post" enctype="multipart/form-data">
<input type="file" name="image" required>
<input type="submit" name="upload" value="Upload File">
</form>
<?php
if( isset($_POST["upload"]) ){
//if form is submitted
// allowed extension array
$allowedExts = array("jpg", "jpeg", "gif", "png");
//Temprory name of file
$tmp_name_of_file = explode(".", $_FILES["image"]["name"]);
//Extension of uploaded file
$extension = end($tmp_name_of_file);
//Checking uploaded file type
//if it is jpeg image, it will print 'image/jpeg'
$file_type = $_FILES["image"]["type"];
// Checking file type and file extension
if( $file_type == "image/gif" || $file_type == "image/jpg" || $file_type == "image/jpeg" || $file_type == "image/png" && in_array($extension, $allowedExts))
{
// file type and extension is OK
// checking if any error in uploaded file
if ($_FILES["image"]["error"] > 0) {
echo "Error: " . $_FILES["image"]["error"];
exit(0);
}else{
// No error in uploaded file, we can move next
/// check mime file content
$verifyimg = getimagesize($_FILES['image']['tmp_name']);
// $verifyimg is an array containing image width , height, mime etc..
//print_r($verifyimg);
if( $verifyimg['mime'] != 'image/png' && $verifyimg['mime'] != 'image/jpg' && $verifyimg['mime'] != 'image/jpeg' && $verifyimg['mime'] != 'image/gif' ) {
echo "Only images are allowed!";
exit(0);
}else{
// image mime checked
// image file type and extension checked
//now check using getimagesize
$image_info = getimagesize($_FILES['image']['tmp_name']); ///////==========
if($image_info == false) {
echo "Not a valid image!";
exit(0);
}else{
// image is also valid
//now, check size
$allowed_size = 100000; //it is in byte// 100000 byte = 100KB
if($_FILES['image']['size'] > $allowed_size){
echo "Allowed size is 100KB ";
exit();
}else{
//Ready to uploaded
$image = $_FILES["image"]["name"];
$image = time().rand(1,888).$image;
// $image variable is file name, you can store this name in database
$tmp_name = $_FILES["image"]["tmp_name"];
move_uploaded_file($tmp_name, "uploads/".$image); ////storage
echo "File Uploaded";
}
}
}
}
}else{
// Invalid File Type and Extension
echo "Invalid image type";
exit(0);
}
} //form submition if end
?>
Download Sample Code